function proyectos_etapas_crud() {
$crud = new grocery_CRUD();
$crud->set_table('fs_etapa');
$crud->set_theme('flexigrid');
$crud->set_subject('Etapas');
$crud->unset_columns('id', 'etapa_nombre_heredado');
$crud->fields('fs_proyecto_id', 'fs_definicion_etapa_id', 'etapa_fecha_inicio', 'etapa_fecha_fin');
$crud->required_fields('etapa_fecha_fin', 'etapa_fecha_inicio');
$crud->set_relation('fs_proyecto_id', 'fs_proyecto', 'proyecto_nombre');
$crud->set_relation('fs_definicion_etapa_id', 'fs_definicion_etapa', 'definicion_etapa_nombre');
$crud->display_as('fs_proyecto_id', 'Proyecto');
$crud->display_as('fs_definicion_etapa_id', 'Etapa');
$crud->display_as('etapa_fecha_inicio', 'Fecha de Inicio');
$crud->display_as('etapa_fecha_fin', 'Fecha de Finalización');
$crud->callback_after_insert(array($this, 'nombre_etapas'));
$crud->callback_after_update(array($this, 'nombre_etapas'));
$crud->callback_before_insert(array($this, 'crear_datos'));
$crud->set_rules('fs_definicion_etapa_id', 'Etapa', 'callback_etapa_check');
$output = $crud->render();
$this->_example_output($output);
}
// Verifica que la etapa no se encuentre ya registrada en el proyecto
public function etapa_check($str) {
if ($this->proyecto_model->etapaProyecto($str, $str['fs_proyecto_id']) == true) {
$this->form_validation->set_message('etapa_check', 'La Etapa ya se encuentra registrada en el Proyecto');
return FALSE;
} else {
return TRUE;
}
}
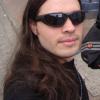
set_rules callback
- Single Page
Posted 28 April 2012 - 15:17 PM
Posted 05 May 2012 - 17:18 PM
Posted 06 May 2012 - 11:27 AM
Posted 01 April 2017 - 00:39 AM
guys... I got a problem.... set_rules() appears to be well stated.. but still not working
I got MY_Form_validation lib
<?php defined('BASEPATH') OR exit('No direct script access allowed'); class MY_Form_validation extends CI_Form_validation { public $CI; /*public function __construct() { $this->CI =& get_instance(); }*/ /*function run($module = '', $group = ''){ (is_object($module)) AND $this->CI =& $module; return parent::run($group); }*/ } /* End of file MY_Form_validation.php */ /* Location: ./application/libraries/MY_Form_validation.php */
and then in the controller call to $this->form_validation->CI =& $this;
but not working.
<?php defined('BASEPATH') OR exit('No direct script access allowed'); class Stock_log extends MY_Controller { function __construct() { parent::__construct(); $this->load->library('template'); //$this->template->add_js('bootstrap/js/bootstrap.min.js'); //$this->load->library('form_validation'); $this->load->library('form_validation'); $this->form_validation->CI =& $this; } public function index() { $crud = new grocery_CRUD(); $crud->set_table('warehouse_stock_log'); $crud->set_subject('Movimiento de Stock'); if (!$this->ion_auth->is_admin()) { $crud->unset_edit(); $crud->unset_delete(); $crud->unset_add_fields('date'); } else{ $crud->unset_add_fields('date'); } $crud->set_relation('item','products_catalog','nombre'); $crud->set_relation('fromm','warehouse_manager','name'); $crud->set_relation('too','warehouse_manager','name'); /* $crud->set_rules('qty', 'qtyy', array( 'requireda', function($value) { // Check $value $this->form_validation->set_message('requireda',"Salt value must be less then FIVE2"); return false; } ) );*/ $crud->set_rules('qty', 'qty','callback_check_qty'); $state = $crud->getState(); $state_info = $crud->getStateInfo(); switch ($state) { case 'list': $crud->set_relation('user','auth_users','{first_name} {last_name}'); break; case 'add': $crud->field_type('user', 'hidden',$this->ion_auth->user()->row()->id); break; default: $crud->set_relation('user','auth_users','{first_name} {last_name}'); break; } $crud->callback_before_insert(array($this,'check2moove_stock')); $crud->callback_after_insert(array($this,'moove_stock')); $output = $crud->render(); //$this->template->set_messages('un mensagesfdgdsf gfdsgfds fdsgdfsgdfsgdfs fddsdsg ds gdfs ds ddsgds dg fds dsd '); //$this->template->set_messages('otro mensage'); //$this->session->set_flashdata('error','Put your error message here'); //$this->session->set_flashdata('error','Put your error message here'); $this->template->template_name('AdminLTE-2.3.7'); $this->template->set_title('Movimiento de Stock'); $this->template->load_view('stock_log',$output); } public function check_qty(){ //$this->load->library('form_validation'); /*$qty = $_POST['qty']; $qty = $str; $this->form_validation->set_message('qty',"Salt value must be less then FIVE"); return false;*/ $this->form_validation()->set_message('check_qty',"Salt value must be less then FIVE1"); $this->form_validation->set_message('check_qty',"Salt value must be less then FIVE2"); //"whatever i write here..... it seems that this function is not found" return FALSE; //die(var_dump($str)); } function stock_log_insert($post_array, $primary_key){ $data['item']=$primary_key; $data['qty']=$post_array['qty']; //$data['date']=$post_array['algo']; $data['user']=$this->ion_auth->user()->row()->id; $data['fromm']=$post_array['from']; $data['too']=$post_array['to']; return $this->db->insert('warehouse_stock_log', $data); } function check2moove_stock($post_array){ $return=false; $ $item_too=$this->db->get_where('warehouse_'.$post_array['too'],array('item'=>$post_array['item']))->result(); $item_fromm=$this->db->get_where('warehouse_'.$post_array['fromm'],array('item'=>$post_array['item']))->result(); if ($item_too!=false and $item_fromm!=false) { if ($item_fromm->real_qty>$post_array['qty'] and ($item_fromm->real_qty-$item_fromm->real_qty)<=$post_array['qty'] ) { return $post_array; } else { // mensaje: la cantidad supera a la cantidad real (o diferencia de virtual) disponible $this->session->set_flashdata('error',"la cantidad supera a la cantidad real (o diferencia de virtual) disponible"); return false; } } else { // mensaje: el producto {postarray['item']} no existe en alguno de los depositos seleccionados. $this->session->set_flashdata('error',"el producto no existe en alguno de los depositos seleccionados."); return false; } if ($return) { return true; } else { return false; } } function moove_stock ($post_array, $primary_key){ /*$this->db->set('real_qty', 'real_qty+'.$post_array['qty'], FALSE); $this->db->set('virtual_qty', 'virtual_qty+'.$post_array['qty'], FALSE); $this->db->where('item', $post_array['item']); if ($this->db->update('warehouse_'.$post_array['too'])) { # code... } else { $this->db->set('real_qty', 'real_qty+'.$post_array['qty'], FALSE); $this->db->set('virtual_qty', 'virtual_qty+'.$post_array['qty'], FALSE); $this->db->set('item', $post_array['item']); $this->db->insert('warehouse_'.$post_array['too']); } $this->db->set('real_qty', 'real_qty-'.$post_array['qty'], FALSE); $this->db->set('virtual_qty', 'virtual_qty-'.$post_array['qty'], FALSE); $this->db->where('item', $post_array['item']); if ($this->db->update('warehouse_'.$post_array['fromm'])) { # code... } else { $this->db->set('real_qty', 'real_qty-'.$post_array['qty'], FALSE); $this->db->set('virtual_qty', 'virtual_qty-'.$post_array['qty'], FALSE); $this->db->set('item', $post_array['item']); $this->db->insert('warehouse_'.$post_array['fromm']); }*/ return $post_array; } } /* End of file Stock_log.php */ /* Location: ./application/modules/deposito/controllers/Stock_log.php */
always the result is : "Unable to access an error message corresponding to your field name qty.(check_qty)
what is wrong?
GC version 1.5.8
* @package grocery CRUD
Posted 01 April 2017 - 00:51 AM
even if I call to an anonymous function
$crud->set_rules('qty', 'qty', array( 'requireda', function($value) { // Check $value $this->form_validation->set_message('requireda',"Salt value must be less then FIVE2"); return false; } ) );
I got: "Unable to access an error message corresponding to your field name qty.(Anonymous function)
"