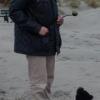
How do i insert a calculated field in a table?
- Single Page
Posted 08 November 2013 - 10:19 AM
I just found out aboud grocery crud, it looks great!
I will defenatly use this, as I am going to write a invoice system.
One question:
How do I manage calculated fields?
Lets say I want to add a record that has these fields:
qty, price, total.
So I enter the qty and price, how do I insert the calculated field "total"?.
Regards Ralph
Posted 09 November 2013 - 06:50 AM
use callback_column
$crud->callback_column('total',array($this,'total'));
function total($value, $row)
{
$quantity = $row->quantity;
$price = $row->price;
$total = $quantity * $price;
return $total;
}
Posted 11 November 2013 - 18:36 PM
Here's an actual example from a program I made. This comes from a master-detail relationship and the code below is from the detail controller. Take note that the calculated field is displayed as a formatted string representing a decimal number with two places.
public function assemble($pk)
Posted 12 November 2013 - 09:24 AM
You can use callback_before_insert to prepare the "total" value to insert into the database
$crud->callback_before_insert(array($this,'calculate')); function calculate($post_array){ $post_array['total'] = $post_array['qty']*$post_array['price']; return $post_array }
Posted 12 November 2013 - 12:29 PM
ok this makes sense,
but what if I have 2 calculated fiels?
do I need to do something like this?
2 times a callback and 2 functions?
$crud->callback_before_insert(array($this,'discount'));
$crud->callback_before_insert(array($this,'total'));
function discount($post_array){
$post_array['total'] = $post_array['qty']*$post_array['price'];
return $post_array
}
function total($post_array){
$post_array['total'] = some code;
return $post_array
}
Posted 12 November 2013 - 12:34 PM
You should use the callback function once.
function callback($post_array){
$post_array['total'] = $post_array['qty']*$post_array['price'];
$post_array['discount'] = some code;
return $post_array
}
for example:
$post_array['total'] = $post_array['qty']*$post_array['price'];
$discount = getting user's discount here;
$post_array['discounted_price'] = $post_array['total']-($post_array['total']*$discount);
end then you will have this result:
$post_array['total'] is 100$
$post_array['discounted_price'] is 80$
Posted 12 November 2013 - 12:42 PM
the callback function have to be used once
Posted 12 November 2013 - 13:05 PM
public function brandstof() { $crud = new grocery_CRUD(); $crud->set_theme('datatables'); $crud->set_table('invoer'); $crud->set_subject('Brandstof'); $crud->required_fields('datum', 'aant_km', 'ltr', 'prijs'); $crud->fields('datum', 'aant_dagen', 'aant_km', 'ltr', 'prijs'); $crud->order_by('datum', 'desc'); $crud->callback_before_insert(array($this,'calc')); $output = $crud->render(); $this->_example_output($output); } function calc($post_array) { $post_array['tot'] = $post_array['prijs'] * $post_array['ltr']; $post_array['een_op'] = $post_array['aant_km'] / $post_array['ltr']; $post_array['ltr_per_100_km'] = $post_array['ltr'] / $post_array['aant_km']; return $post_array; }
Posted 12 November 2013 - 13:06 PM
show your table structure
Posted 12 November 2013 - 13:09 PM
i asume you mean the SQL table?
se attached tumbnail[attachment=710:table structure.jpg]
Posted 12 November 2013 - 13:16 PM
re: the result is that he's not entering the calculated fields.
is the field is empty after inserting?
make print_r($post_array);
an you will see the post result
Posted 12 November 2013 - 13:27 PM
in phpmyadmin I see null in the calculated fields
make print_r($post_array);
an you will see the post result
yes about that
so I edit this record and hit save
where do I put this print_r($post_array) ??
in my main function or view?
Posted 12 November 2013 - 14:30 PM
the strange thing is that phpmyadmin displays null in the calculated field
and if I don't call
$crud->callback_before_insert(array($this,'calc'));