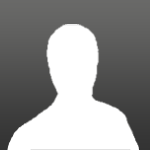
How do I add date/time to file name instead of random prefix?
- Single Page
Posted 19 December 2012 - 15:08 PM
Posted 19 December 2012 - 16:01 PM
private function trim_file_name($name, $type)
{
$file_name = trim(basename(stripslashes($name)), ".\x00..\x20");
if (strpos($file_name, '.') === false && preg_match('/^image\/(gif|jpe?g|png)/', $type, $matches))
{
$file_name .= '.' . $matches[1];
}
$file_name = date('d-m-y-H-i-s',time()).'.'.substr(strrchr($file_name,'.'), 1);
return $file_name;
}
Result: 19-12-2012-18-29-32.jpg
Posted 19 December 2012 - 19:53 PM
for example:
private function trim_file_name($name, $type)
{
$file_name = trim(basename(stripslashes($name)), ".\x00..\x20");
if (strpos($file_name, '.') === false && preg_match('/^image\/(gif|jpe?g|png)/', $type, $matches))
{
$file_name .= '.' . $matches[1];
}
$file_name = date('d-m-y-H-i-s',time()).'.'.substr(strrchr($file_name,'.'), 1);
return $file_name;
}
Result: 19-12-2012-18-29-32.jpg
[/quote]
That was helpful. Do I simply replace the code in the file "grocery_crud.php" with my own code? I could not see how to write an extension to this class because the function is private.
After reviewing your example, I wrote the following to better match my intent.
private function trim_file_name($name, $type)
{
// Remove path information and dots around the filename, to prevent uploading
// into different directories or replacing hidden system files.
// Also remove control characters and spaces (\x00..\x20) around the filename:
$file_name = trim(basename(stripslashes($name)), ".\x00..\x20");
// get filename extension
$extension = pathinfo($file_name, PATHINFO_EXTENSION);
// get base filename
$filename = pathinfo($file_name, PATHINFO_FILENAME);
// combine filename parts with timestamp added after base filename
$file_name = $filename.'-'.date('YmdHis',time()).'.'.$extension;
return $file_name;
}
Are there potential problems with my approach?
Can I omit this line since I am getting the base filename and extension from pathinfo()?
$file_name = trim(basename(stripslashes($name)), ".\x00..\x20");
Posted 21 December 2012 - 11:42 AM
Do I simply replace the code in the file "grocery_crud.php" with my own code?
[/quote]
Yes
[quote]
Can I omit this line since I am getting the base filename and extension from pathinfo()?
[/quote]
undesirable
In your case the filename can include spaces and other illegal characters. You should to validate the filename.
Posted 21 December 2012 - 17:25 PM
https://www.owasp.org/index.php/XSS_Filter_Evasion_Cheat_Sheet
Posted 21 December 2012 - 18:14 PM
I think this article will be useful for you and for other people:
https://www.owasp.or...ion_Cheat_Sheet
[/quote]
That is a very interesting site. I read the following first:
[quote]
Tip: if you're in a rush and need to quickly check a page, often times injecting the depreciated "<PLAINTEXT>" tag will be enough to check to see if something is vulnerable to XSS by messing up the output appreciably:
[/quote]
I was surprised that entering that tag in GroceryCRUD add forms resulted in putting very unexpected code in my database? That would appear to be a major bug and vulnerability.
I am still rather inexperienced with such things, so I would appreciate an explanation.
Posted 21 December 2012 - 18:31 PM
because the grocery crud library doesn't know what data you want save.
Sorry my English isn't good enough.
Posted 21 December 2012 - 18:34 PM
Posted 22 December 2012 - 01:03 AM
$config['global_xss_filtering'] = TRUE;
After making that change, entering the tag in the form just passes the tag as it was typed to the database.
Posted 22 December 2012 - 08:40 AM
$this->input->post('some_data', TRUE);- it's the best solution for me.
If you want to delete all or some tags from the post data too you can use the "strip_tags":
$data = strip_tags($this->input->post('some_data', TRUE));
Posted 04 January 2013 - 18:51 PM
The modified method would be:
private function trim_file_name($name, $type) {
$name = trim(basename(stripslashes($name)), ".\x00..\x20");
if (strpos($file_name, '.') === false &&
preg_match('/^image\/(gif|jpe?g|png)/', $type, $matches)) {
$extension = '.'.$matches[1];
}
$file_name = substr(uniqid(),-8).$extension;
return $file_name;
}
thanks
Posted 07 August 2013 - 09:25 AM
can i add default date and time in the data base in new fileds
Posted 07 August 2013 - 18:04 PM
can i add default date and time in the data base in new fileds
I am not sure I understand the question.
Have you seen this code?
Modify it to your requirements.
Posted 08 August 2013 - 02:32 AM
i want to date and time in data base as the time on creation as the new filed in data base